What is CSRF token ? how to implement CSRF token in html form?
Posted By: Vaishnavi Mall Published: 14, Jan 2024
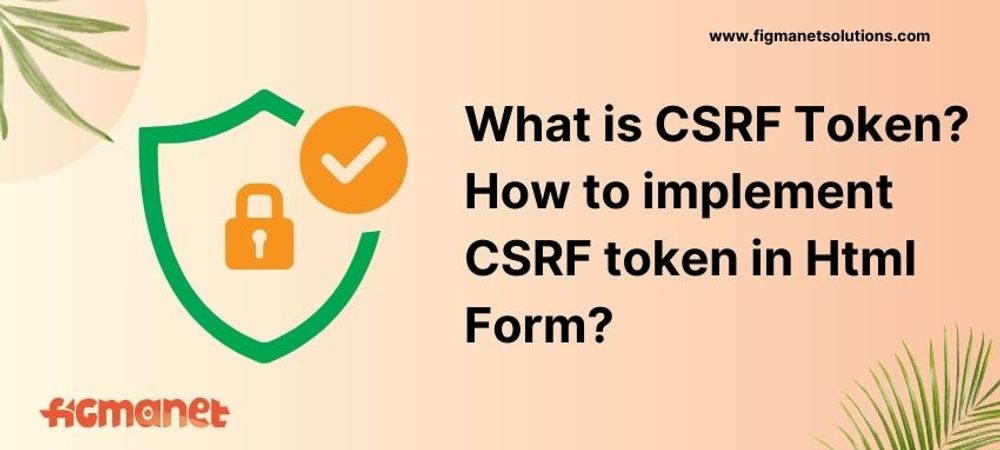
Introduction
CSRF stands for Cross-Site Request Forgery which means committing a crime by making an illegal cross-site request but what it is a cross-site request. There are two types of requests that can be made on a website.
- Same site request: When a webpage from a website sends an HTTP request to the same website, it is the same site request.
- Cross-site request: When a webpage from a website sends an HTTP request to a different website, it is a cross-site request.
- When a request coming from facebook.com is sent to facebook.com the browser attaches all the cookies belonging to facebook.com and when a request comes from any other website is sent to Facebook.com the browser still attaches all the cookies belonging to facebook.com to the request.
- As a result of this behavior of the browser, the server fails to distinguish between the same site request and cross-site request.
- Hence it is easy for third-party websites to forge requests that are the same as the same site request. This is called Cross-Site Request Forgery.
- Use a random number generator for generating the token.
- Expire the tokens after a short duration of time so that they can’t be reused.
- Do not send CSRF tokens in HTTP to prevent them from being directly available in the URL.
- CSRF tokens should never be transmitted using Cookies.
Cross-Site Request Forgery
CSRF tokens are a great mechanism for protection against CSRF attacks.
What are CSRF tokens?
A CSRF token is a unique, secret unpredictable alphanumeric value that is generated by the server and transmitted to the client-side in such a way that is included in all subsequent requests made by the client in order to protect CSRF's vulnerable resources from CSRF attacks.
After the generation of the token in the subsequent request made, the request is checked for the expected token if the token is missing or invalid the request is rejected.
Generating CSRF tokens
CSRF tokens should be made very secure, secret, unpredictable, and with a very short life so that they can't be used again and again.
In PHP a token can be made like this :
$_SESSION[‘token’] = bin2hex(random_bytes(100));
And then can be verified like this
if (hash_equals($_SESSION[‘token’], $_POST[‘token’])) {
...
} else {
...
}
Transmitting CSRF tokens
CSRF tokens should be handled very carefully throughout their lifecycle. They can be transmitted in HTML forms as hidden fields. They should be placed as early as possible in the form before any input field. Then the hidden field will be included in the request parameter when the form is submitted.
For example
Validating CSRF Tokens
When a CSRF token is generated it should be saved in the user’s session data on the server-side. When a subsequent request is generated which needs to be validated then the server should check for the token in the request. If the request is missing the token or the token present is invalid the request should be rejected.